Hello Guys! In this blog, I’m going to explain to you how to create RGBA Color Generator using JavaScript. This will be a step-by-step guide including HTML and CSS. Let’s get started 🚀.
Before we start, here are some more JavaScript Games you might like to create:
1. Snake Game using JavaScript
2. 2D Bouncing Ball Game using JavaScript
3. Rock Paper Scissor Game using JavaScript
4. Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeÂ
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- Font Awesome Icons --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA==" crossorigin="anonymous" /> <!-- Google Fonts --> <link rel="preconnect" href="https://fonts.googleapis.com"> <link rel="preconnect" href="https://fonts.gstatic.com" crossorigin> <link href="https://fonts.googleapis.com/css2?family=Poppins&display=swap" rel="stylesheet"> <title>RGBA Color Generator - @code.scientist x @codingtorque</title> </head> <body> <div class="card"> <div class="sliders"> <label for="red">Red</label> <input type="range" min="0" max="255" value="0" oninput="generateRGBA()" id="red"> <label for="green">Green</label> <input type="range" min="0" max="255" value="0" oninput="generateRGBA()" id="green"> <label for="blue">Blue</label> <input type="range" min="0" max="255" value="0" oninput="generateRGBA()" id="blue"> <label for="alpha">Aplha</label> <input type="range" min="0.1" max="1.0" step="0.1" value="1" oninput="generateRGBA()" id="alpha"> </div> <div class="output-box"> <div class="output" id="output">rgba(0,0,0,1)</div> <div class="copy-btn" onclick="copy()"><i class="fas fa-copy"></i></div> </div> </div> </body> </html>
Output Till Now
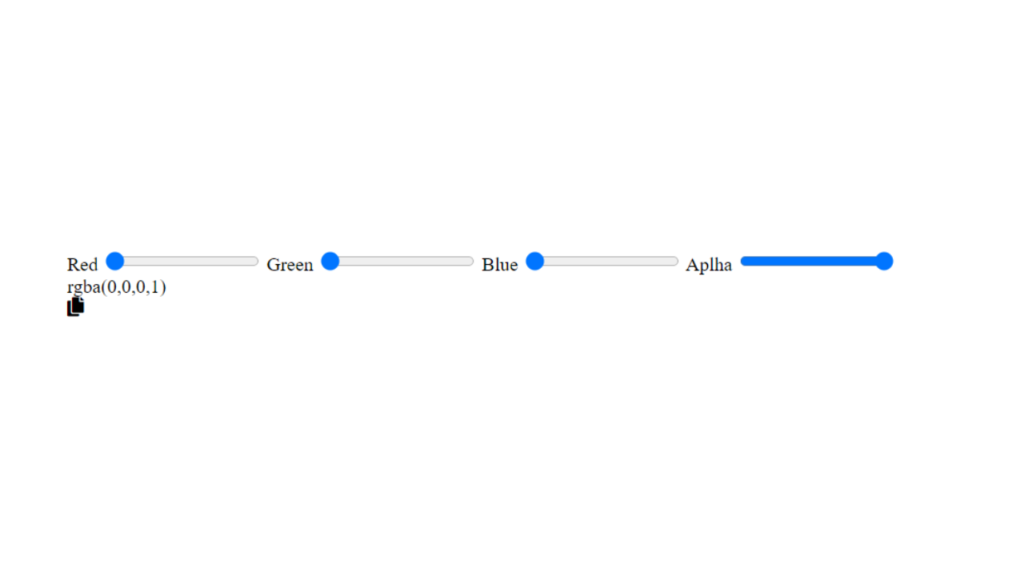
CSS CodeÂ
Create a file style.css
and paste the code below.
* { font-family: 'Poppins', sans-serif; } body { background-color: #111827; color: white; display: flex; align-items: center; justify-content: center; } .card { width: 15rem; border-radius: 10px; overflow: hidden; background: #1f2937; padding: 30px 20px; } .sliders { display: flex; flex-direction: column; } .output-box { display: flex; align-items: center; width: fit-content; margin-top: 1rem; } .output { border: 2px solid; padding: 6px 20px; } .copy-btn { cursor: pointer; padding: 12px; background: #111827; }
Output Till Now
JavaScript CodeÂ
Create a fileÂ
script.js
 and paste the code below.const generateRGBA = () => { let red = document.getElementById("red").value; let green = document.getElementById("green").value; let blue = document.getElementById("blue").value; let alpha = document.getElementById("alpha").value; let output = document.getElementById("output"); output.innerText = `rgba(${red}, ${green}, ${blue}, ${alpha})` output.style.background = `rgba(${red}, ${green}, ${blue}, ${alpha})` } const copy = () => { let output = document.getElementById("output"); navigator.clipboard.writeText(output.innerText) alert('Copied') }
Written by: Piyush Patil
If you have any doubts or any project ideas feel free to Contact Us
Hope you find this post helpful💖