Hello fellow coders! Welcome to Coding Torque, where we explore the exciting world of coding through hands-on projects and tutorials. In today’s blog post, we will be diving into the creation of a GIF searcher application using HTML, CSS, and JavaScript. This app will utilize the power of the Giphy API to retrieve GIFs based on user-specified keywords, making it easy for you to find the perfect GIF for any occasion. So grab your favorite beverage and let’s get started!
Before we start, here are some JavaScript Games you might like to create:
1.Ā Snake Game using JavaScript
2.Ā 2D Bouncing Ball Game using JavaScript
3.Ā Rock Paper Scissor Game using JavaScript
4.Ā Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeĀ
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- CSS --> <link rel="stylesheet" href="style.css"> <!-- Fontawesome Icons CDN --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.14.0/css/all.min.css" integrity="sha512-1PKOgIY59xJ8Co8+NE6FZ+LOAZKjy+KY8iq0G4B3CyeY6wYHN3yt9PW0XpSriVlkMXe40PTKnXrLnZ9+fkDaog==" crossorigin="anonymous" /> <title>GIF Search uisng GIPHY API using JavaScript - @code.scientist x @codingtorque</title> </head> <body> <!-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag
<header className='header'> <h1 className='header__heading'> <span className='header__logo'>GIPHY</span> <span>Search API</span> </h1> </header> <div class="search"> <input type="text" class="input userinput" placeholder="Search..."> <button class="btn searchBtn"> <i class="fas fa-search"></i> </button> </div> <div class="container gif-results"> </div> <div id="giphy-search"></div> <footer className='footer'> <a className='footer__link' href='https://developers.giphy.com/' aria-label='GIPHY Developers' target='_blank'> <img className='footer__image' src='http://www.georgewpark.com/images/codepen/giphy-attribution.png' alt='Powered By GIPHY' /> </a> </footer>
Output Till Now
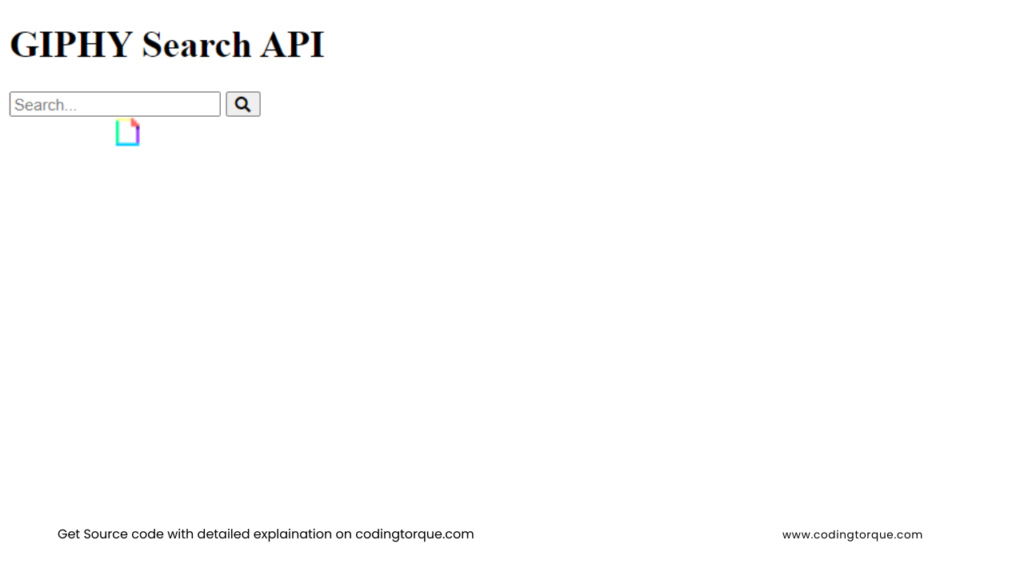
CSS CodeĀ
Create a fileĀ style.css
and paste the code below.
:root { --black: #111; --grey: #222; --white: #fcfcfc; } * { box-sizing: border-box; } body { font-family: "Open Sans", Arial, sans-serif; min-height: 100vh; color: var(--white); background-color: var(--grey); text-align: center; } .container { max-width: 62.5rem; margin: 0 auto; padding: 2rem 1.25rem 7rem; } /* Header */ .header { padding: 1.8rem 1.25rem; background-color: var(--black); } .header__heading { font-size: calc(1.8rem + 1vw); font-weight: 300; line-height: 1.4; } .header__heading span { white-space: nowrap; } .header__logo { font-weight: 800; } /* Search */ .search { display: flex; align-items: center; justify-content: center; height: 50px; } .search .input { background-color: #fff; border: 0; font-size: 18px; padding: 15px; height: 50px; width: 200px; } .btn { background-color: #fff; border: 0; cursor: pointer; font-size: 24px; height: 50px; width: 50px; transition: transform 0.3s ease; } .btn:focus, .input:focus { outline: none; } .search.active .btn { transform: translateX(198px); } /* Results */ .gif-results { display: grid; grid-template-columns: repeat(auto-fit, minmax(12rem, 1fr)); gap: 1.8rem; align-items: center; font-size: 1.6rem; } .gif { animation: fadeInUp 1s; } .gif__image { width: 100%; height: 100%; object-fit: cover; box-shadow: 0 0.2rem 0.4rem rgba(0, 0, 0, 0.16), 0 0.3rem 0.4rem rgba(0, 0, 0, 0.23); } /* Footer */ .footer { display: flex; justify-content: center; position: fixed; bottom: 0; width: 100%; z-index: 1; padding: 1.4rem 1.25rem; background-color: var(--black); } .footer__image { max-width: 100%; } /* Loader Animation */ @keyframes loader { to { transform: rotate(1turn); } } /* Fade Up Animation */ @keyframes fadeInUp { from { opacity: 0; transform: translateY(50%); } to { opacity: 1; transform: translateY(0); } }
Output Till Now
JavaScript CodeĀ
script.js
and paste the code below.function getUserInput() { var inputValue = document .querySelector(".userinput").value; return inputValue; } document.querySelector(".searchBtn").addEventListener("click", function () { var inputValue = document .querySelector(".userinput").value; var userInput = getUserInput(); searchGiphy(userInput); }); document.querySelector(".userinput") .addEventListener("keyup", function (e) { if (e.which === 13) { var userInput = getUserInput(); searchGiphy(userInput); } }); function searchGiphy(searchQuery) { var url = "https://api.giphy.com/v1/gifs/search?api_key=YOUR_API_KEY&q=" + searchQuery; var GiphyAJAXCall = new XMLHttpRequest(); GiphyAJAXCall.open("GET", url); GiphyAJAXCall.send(); GiphyAJAXCall.addEventListener("load", function (data) { var actualData = data.target.response; pushToDOM(actualData); console.log(actualData); }); } function pushToDOM(response) { response = JSON.parse(response); var images = response.data; var container = document.querySelector(".gif-results"); container.innerHTML = ""; images.forEach(function (image) { var src = image.images.fixed_height.url; container.innerHTML += "<img src='" + src + "' class='gif gif__image' />"; }); }