Hello Guys! In this blog, I’m going to explain to you how to create Loan calculator using JavaScript. This will be a step-by-step guide including HTML and CSS. Let’s get started 🚀.
Before we start, here are some more JavaScript Games you might like to create:
1. Snake Game using JavaScript
2. 2D Bouncing Ball Game using JavaScript
3. Rock Paper Scissor Game using JavaScript
4. Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeÂ
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- Font Awesome Icons --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA==" crossorigin="anonymous" /> <!-- Google Fonts --> <link rel="preconnect" href="https://fonts.googleapis.com"> <link rel="preconnect" href="https://fonts.gstatic.com" crossorigin> <link href="https://fonts.googleapis.com/css2?family=Poppins&display=swap" rel="stylesheet"> <title>Loan Calculator - @code.scientist x @codingtorque</title> </head> <body> <div class="container"> <h1>Loan Calculator</h1> <div class="form-input"> <button class="input-icon"><i class="fas fa-dollar-sign"></i></button> <input type="number" class="input" id="amount" placeholder="Loan Amount"> </div> <div class="form-input"> <button class="input-icon"><i class="fas fa-percentage"></i></button> <input type="number" class="input" id="interest" placeholder="Interest"> </div> <div class="form-input"> <button class="input-icon"><i class="fas fa-calendar"></i></button> <input type="number" class="input" id="months" placeholder="Months to repay"> </div> <button class="calculateBtn" onclick="calculateLoan()">Calculate</button> <div id="result"></div> </div> </body> </html>
Output Till Now
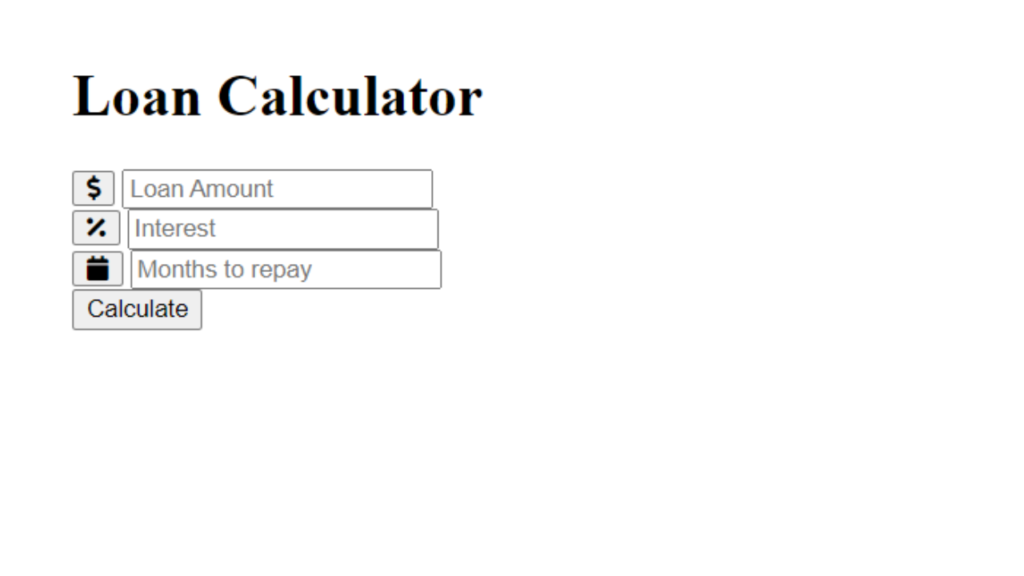
CSS CodeÂ
Create a file style.css
and paste the code below.
* { font-family: 'Poppins', sans-serif; } body { background-color: #111827; color: white; display: flex; align-items: center; justify-content: center; } .container { display: flex; flex-direction: column; align-items: center; border: 2px solid deepskyblue; padding: 40px; border-radius: 20px; width: 30rem; } .form-input { border: 2px solid deepskyblue; width: 14rem; height: 2rem; background: white; border-radius: 5px; margin: 5px 0; } .input { height: 100%; width: 80%; border: none; outline: none; padding: 0 10px; } .input-icon { width: 15%; height: 100%; border: none; cursor: pointer; } .calculateBtn { height: 3rem; width: 8rem; border: 2px solid deepskyblue; background: deepskyblue; color: white; font-size: 16px; border-radius: 7px; padding: 8px; margin-top: 10px; cursor: pointer; }
Output Till Now
JavaScript CodeÂ
Create a fileÂ
script.js
 and paste the code below.const calculateLoan = () => { let amount = document.getElementById("amount").value; let interest = document.getElementById("interest").value; let months = document.getElementById("months").value; let monthlyPayment = ((amount / months) + interest); let totalInterest = (amount * (interest * 0.01)) / months; let totalPayment = parseFloat(amount) + parseFloat(totalInterest); document.getElementById("result").innerHTML = ` <h2>Results : </h2> <h4>Total payment : ${parseFloat(totalPayment).toFixed(2)}</h4> <h4>Monthly Payment : ${parseFloat(monthlyPayment).toFixed(2)}</h4> <h4>Total Interest : ${parseFloat(totalInterest).toFixed(2)}</h4>`; }
Written by: Piyush Patil
If you have any doubts or any project ideas feel free to Contact Us
Hope you find this post helpful💖