Clocks are a fundamental part of our daily lives, and designing one that is both functional and visually appealing can be an exciting challenge. In this tutorial, we’ll show you how to create a “Pie Time Clock” using HTML, CSS, and JavaScript. This clock design features a unique circular “pie” chart that displays the hours, minutes, and seconds of the day. We’ll guide you through the process of building the clock’s HTML structure and then show you how to use CSS to style it, giving it a modern and sleek appearance. We’ll also demonstrate how to use JavaScript to make the clock functional, with the pie chart updating in real-time as time passes. By the end of this tutorial, you’ll have a fully-functional and aesthetically pleasing clock that will impress anyone who sees it. So, let’s dive into creating a “Pie Time Clock” using HTML, CSS, and JavaScript!
Before we start here are 50 projects to create using HTML CSS and JavaScript –
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
Demo
HTML Code
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Pie Time Clock using HTML CSS and JavaScript - Coding Torque</title> </head> <body> <!-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag.
<canvas id="canv" width="500" height="500"></canvas>
CSS Code
Create a file style.css and paste the code below.
@import url(https://fonts.googleapis.com/css?family=Noto+Serif:700); body { margin: 0; background: hsla(180, 95%, 15%, 1); font-family: "Noto Serif", serif; font-size: 4em; overflow: hidden; width: 100%; } canvas { left: 50%; position: absolute; top: 50%; transform: translate(-50%, -50%); }
JavaScript Code
Create a file script.js and paste the code below.
var c = document.getElementById('canv'); var $ = c.getContext('2d'); var ang = 0; var secondsColor = 'hsla(180, 85%, 5%, .7)'; var minutesColor = 'hsla(180, 95%, 15%, 1)'; var hoursColor = 'hsla(180, 75%, 25%, 1)'; var currentHr; var currentMin; var currentSec; var currentMillisec; var t = setInterval('updateTime()', 50); function updateTime() { var currentDate = new Date(); var g = $.createRadialGradient(250, 250, .5, 250, 250, 250); g.addColorStop(0, 'hsla(180, 55%, 8%, 1)'); g.addColorStop(1, 'hsla(180, 95%, 15%, 1)'); $.fillStyle = g; $.fillRect(0, 0, c.width, c.height); currentSec = currentDate.getSeconds(); currentMillisec = currentDate.getMilliseconds(); currentMin = currentDate.getMinutes(); currentHr = currentDate.getHours(); if (currentHr == 00) { //if midnight (00 hours) hour = 12 currentHr = 12; } else if (currentHr >= 13) { //convert military hours at and over 1300 (1pm) to regular hours by subtracting 12. currentHr -= 12; } drawSeconds(); drawMinutes(); drawHours(); var realTime = currentHr + ':' + numPad0(currentMin) + ':' + numPad0(currentSec); /*Here is the selected option of creating the text within the pie canvas elemenet */ var textPosX = 250 - ($.measureText(realTime).width / 2); $.shadowColor = 'hsla(180, 100%, 5%, 1)'; $.shadowBlur = 100; $.shadowOffsetX = 12; $.shadowOffsetY = 0; $.fillStyle = 'hsla(255,255%,255%,.7)'; $.font = "bold 1.6em 'Noto Serif', serif"; $.fillText(realTime, textPosX, c.height / 2 + 50); /* OR using a div to display the time (#time) where I pre-styled text with a long shadow using css...can't decide which I like better - but since this is a canvas demo....; (comment out the above text settings and uncomment the below) document.getElementById('time').innerHTML = realTime; */ } function drawSeconds() { ang = 0.006 * ((currentSec * 1000) + currentMillisec); $.fillStyle = secondsColor; $.beginPath(); $.moveTo(250, 250); $.lineTo(250, 50); $.arc(250, 250, 200, calcDeg(0), calcDeg(ang), false); $.lineTo(250, 250); $.shadowColor = 'hsla(180, 45%, 5%, .4)'; $.shadowBlur = 15; $.shadowOffsetX = 15; $.shadowOffsetY = 15; $.fill(); } function drawMinutes() { ang = 0.0001 * ((currentMin * 60 * 1000) + (currentSec * 1000) + currentMillisec); $.fillStyle = minutesColor; $.beginPath(); $.moveTo(250, 250); $.lineTo(250, 100); $.arc(250, 250, 150, calcDeg(0), calcDeg(ang), false); $.lineTo(250, 250); $.shadowColor = 'hsla(180, 25%, 5%, .4)'; $.shadowBlur = 15; $.shadowOffsetX = 15; $.shadowOffsetY = 15; $.fill(); } function drawHours() { ang = 0.000008333 * ((currentHr * 60 * 60 * 1000) + (currentMin * 60 * 1000) + (currentSec * 1000) + currentMillisec); if (ang > 360) { ang -= 360; } $.fillStyle = hoursColor; $.beginPath(); $.moveTo(250, 250); $.lineTo(250, 150); $.arc(250, 250, 100, calcDeg(0), calcDeg(ang), false); $.lineTo(250, 250); $.shadowColor = 'hsla(180, 45%, 5%, .4)'; $.shadowBlur = 15; $.shadowOffsetX = 15; $.shadowOffsetY = 15; $.fill(); } function calcDeg(deg) { return (Math.PI / 180) * (deg - 90); } //handle zeros for minutes and seconds function numPad0(str) { var cStr = str.toString(); if (cStr.length < 2) { str = 0 + cStr; } return str; } window.addEventListener('resize', function () { c.width = 500; c.height = 500; });
Final Output
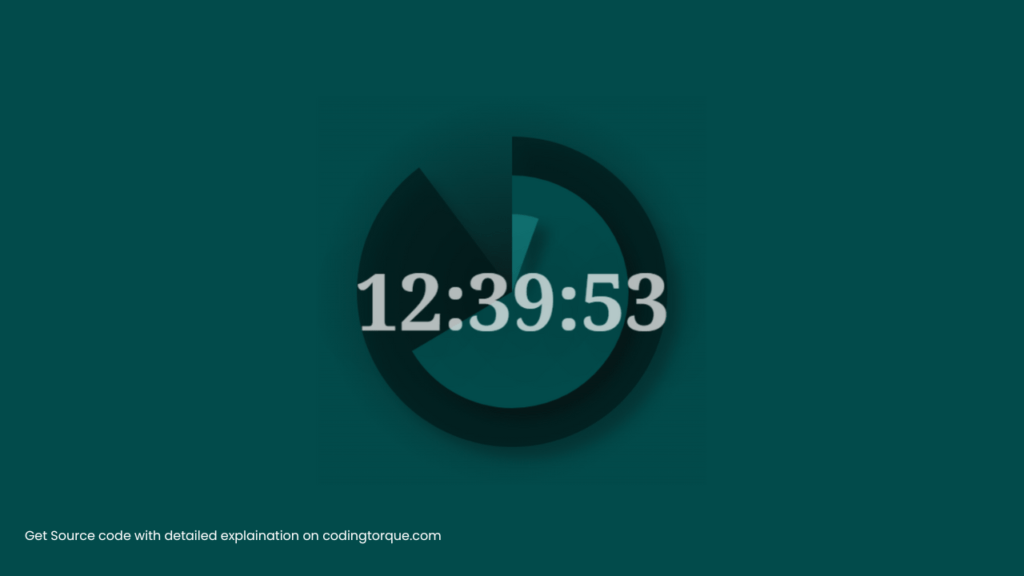
Written by: Piyush Patil
Code Credits: @tmrDevelops
If you found any mistakes or have any doubts please feel free to Contact Us
Hope you find this post helpful💖