Greetings, fellow coders! Are you ready to put your HTML, CSS, and JavaScript skills to the test by creating an engaging and challenging word guessing game? In this blog post, we will take a deep dive into the process of developing a Word Guessing Game using these powerful web development tools. Not only will you get to apply your knowledge of HTML, CSS, and JavaScript, but you will also learn about game logic, user interaction, and how to create an engaging user experience. So, get ready to flex your coding muscles and join me on this exciting journey of building a Word Guessing Game from scratch!
Before we start here are some more projects you might like to create –
- Gallery hover effect using HTML and CSS
- Text with hover effect using pure css
- Team Profile with hover effect using HTML and CSS
- Cards with hover effect using HTML and CSS
- Ying & Yang Cats with hover effect using HTML and CSS
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML Code
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- Bootstrap CSS --> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous"> <!-- Font Awesome Icons --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA==" crossorigin="anonymous" /> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Guess the Word Game using JavaScript - Coding Torque</title> </head> <body class="bg-dark text-white"> <!-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag.
<div id="chooseDifficulty" style="display: block"> <h1>Welcome to Word Guessing Game</h1> <h5 class="my-4">Choose a difficulty</h5> <button type="button" onclick="chooseDif1()" class="btn btn-secondary btn-lg px-4 mx-2">Easy</button> <button type="button" onclick="chooseDif2()" class="btn btn-success btn-lg px-4 mx-2">Normal</button> <button type="button" onclick="chooseDif3()" class="btn btn-danger btn-lg px-4 mx-2">Hard</button> </div> <div id="startButton" style="display: none"> <h1>Guess a Word</h1> <button class="btn btn-success btn-lg px-4 mx-2" onclick="start()">Start</button> <p>Note: Some words may contain a hyphen(-) ex: X-ray</p> </div> <div id="mainGame" style="display: none"> <p>Enter your guess</p> <input type="text" placeholder="A" id="guess" maxlength="1"> <div> <button onclick="enterGuess()" class="btn btn-primary my-2">Enter Guess</button> </div> </div> <div id="RRguess" style="display: block"> <p id="rightGuess"></p> <p id="wrongGuess"></p> <p id="guessesLeft"></p> </div> <div id="youLose" style="display: none"> <h1>You Lose</h1> <div> <button onclick="restart()">Play again?</button> </div> <p id="correctWordWas"></p> </div> <div id="youWin" style="display: none"> <h2>You Win</h2> <div> <button onclick="restart()">Play again?</button> </div> </div>
CSS Code
Create a file style.css and paste the code below.
body { background-size: 100%; position: absolute; top: 50%; left: 50%; transform: translateX(-50%) translateY(-50%); text-align: center; } #guess { height: 50px; width: 50px; border-radius: 10px; border: none; outline: none; text-align: center; font-size: 24px; text-transform: uppercase; } #rightGuess { display: flex; text-transform: uppercase; } #wrongGuess { display: flex; align-items: center; text-transform: uppercase; } .wordBlocks, .wrongWordBlocks { height: 50px; width: 50px; margin-right: 10px; display: flex; justify-content: center; align-items: center; font-size: 26px; background: white; color: black; border-radius: 10px; } .wrongWordBlocks { background: #dc3545; color: white; }
Output Till Now
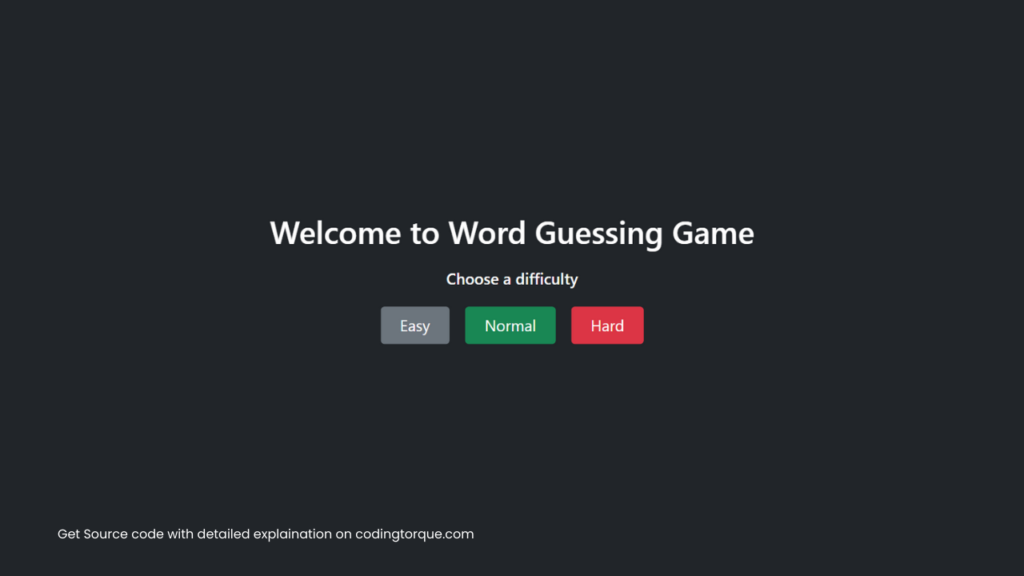
JavaScript Code
Create a file script.js and paste the code below.
var word = ""; var wordGuess = []; var wrongGuess = []; var guessBomb = 0; var winCount = 1; var guess = ""; var dif = 0; function chooseDif1() { dif = 1; document.getElementById('startButton').style.display = 'block'; document.getElementById('chooseDifficulty').style.display = 'none'; } function chooseDif2() { dif = 2; document.getElementById('startButton').style.display = 'block'; document.getElementById('chooseDifficulty').style.display = 'none'; } function chooseDif3() { dif = 3; document.getElementById('startButton').style.display = 'block'; document.getElementById('chooseDifficulty').style.display = 'none'; } function wordw() { var randomWords = ["humor", "miniature", "amusing", "creepy", "fact", "risk", "verse", "land", "lumpy", "holiday", "glorious", "weigh", "brake", "pretty", "grin", "capricious", "bite-sized", "misty", "ignore", "certain", "sloppy", "dress", "true", "zonked", "observation", "action", "various", "want", "direful", "suck", "dress", "scarecrow", "judge", "madly", "quizzical", "consist", "fierce", "love", "arrest", "serve", "fit", "hug", "tan", "curve", "eatable", "tub", "race", "innocent", "open", "preach", "steady", "acoustics", "lock", "field", "arrange", "rifle", "learned", "toe", "flow", "competition", "ill-fated", "oatmeal", "match", "male", "measure", "loaf", "smile", "wrestle", "dull", "food", "locket", "bell", "beg", "strengthen", "responsible", "enchanting", "loutish", "switch", "idea", "nine", "squeamish", "pig", "bat", "dear", "trains", "owe", "frogs", "assorted", "lonely", "hurry", "natural", "sun", "snow", "obnoxious", "broken", "friend", "bright", "cake", "sour", "permit", "economic", "lovely", "quick", "van", "tempt", "apparel", "decay", "business", "adjustment", "blushing", "makeshift", "slippery", "load", "winter", "exist", "tongue", "country", "roll", "fast", "moor", "possess", "pat", "pass", "books", "impartial", "hospitable", "dust", "naughty", "extra-large", "tacky", "produce", "committee", "fuzzy", "judicious", "nebulous", "stick", "ear", "copy", "friendly", "press", "distinct", "vegetable", "upset", "venomous", "statement", "sulky", "spell", "x-ray", "square", "taste", "great", "thumb", "adjoining", "chilly", "test", "ancient", "green", "badge", "work", "repeat", "free", "elderly", "doctor", "difficult", "grubby", "approval", "turn", "vivacious", "thundering", "cherries", "rest", "plan", "crime", "sticks", "wealthy", "phone", "suspend", "gullible", "fence", "note", "wall", "interest", "coil", "jump", "enchanted", "funny", "racial", "greasy", "polish", "elbow", "smart", "bore", "crowd", "glistening", "oval", "eggs", "nauseating", "detailed", "veil", "coal"] var raNum = Math.floor(Math.random() * 70); return randomWords[raNum] } function wordStart() { var wordLength = word.length; var wordL_ = ""; var count = wordLength; while (count > 0) { wordGuess.push("<div class='wordBlocks'></div>"); count -= 1; } } function winCountFunc() { var num = 0; var lettUsed = ""; var count = word.length; while (count > 0) { if (lettUsed.includes(word[count - 1])) { } else { num += 1; lettUsed += word[count - 1]; } count -= 1; } return num; } function start() { word = wordw(); winCount = winCountFunc(); if (dif == 1) { guessBomb = word.length + 5; } else if (dif == 2) { guessBomb = word.length; } else if (dif == 3) { if (word.length % 2 == 0) { guessBomb = word.length / 2; } else { guessBomb = (word.length - 1) / 2; } } console.log(word); document.getElementById('mainGame').style.display = 'block'; document.getElementById('startButton').style.display = 'none'; wordStart(); document.getElementById('RRguess').style.display = 'block'; document.getElementById("rightGuess").innerHTML = wordGuess.toString().replaceAll(',', ' '); document.getElementById("wrongGuess").innerHTML = "Wrong guesses: " + wrongGuess; document.getElementById("guessesLeft").innerHTML = "Guesses remaining: " + guessBomb; var x = document.getElementById("guess").maxLength; } function enterGuess() { var lett = document.getElementById("guess").value; document.getElementById("guess").value = ""; if (lett.length === 1) { var rightOnot = isRightOnot(lett); if (rightOnot == true) { NewCW(lett); } else { if (!wrongGuess.includes(lett)) { wrongGuess.push(`<div class='wrongWordBlocks'>${lett.toString().replaceAll(',', ' ')}</div>`); } guessBomb -= 1; } } else if (lett.length < 1) { } else { guessBomb -= 1; } if (guessBomb <= 0) { gameLose() } if (winCount <= 0) { gameWin() } document.getElementById("rightGuess").innerHTML = wordGuess.toString().replaceAll(',', ' '); document.getElementById("wrongGuess").innerHTML = "Wrong guesses: " + wrongGuess; document.getElementById("guessesLeft").innerHTML = "Guesses remaining: " + guessBomb; } function isRightOnot(a) { var n = word.includes(a); return n; } function NewCW(letter) { var count = 0; winCount -= 1 while (count <= word.length - 1) { if (letter === word[count]) { if (wordGuess[count] === letter) { } else { } wordGuess[count] = `<div class='wordBlocks'>${letter}</div>`; count += 1; } else { count += 1; } } } function gameLose() { document.getElementById('mainGame').style.display = 'none'; document.getElementById('RRguess').style.display = 'none'; document.getElementById('youLose').style.display = 'block'; document.getElementById("correctWordWas").innerHTML = "The correct word was " + word; } function gameWin() { document.getElementById('mainGame').style.display = 'none'; document.getElementById('RRguess').style.display = 'none'; document.getElementById('youWin').style.display = 'block'; } function restart() { document.getElementById('mainGame').style.display = 'none'; document.getElementById('RRguess').style.display = 'none'; document.getElementById('youLose').style.display = 'none'; document.getElementById('youWin').style.display = 'none'; document.getElementById('chooseDifficulty').style.display = 'block'; word = ""; wordGuess = []; wrongGuess = []; guessBomb = 0; winCount = 1; guess = ""; dif = 0; }
Written by: Piyush Patil
If you found any mistakes or have any doubts please feel free to Contact Us
Hope you find this post helpful💖