Welcome to Coding Torque. In this blog, we are going to create a Random Quote Generator using HTML, CSS & JavaScript. You should create this project if you are a beginner and learning JavaScript. In this app, I have simply used an API to fetch quotes randomly and displayed them using JavaScript DOM functions.
Before we start, here are some JavaScript Games you might like to create:
1.Ā Snake Game using JavaScript
2.Ā 2D Bouncing Ball Game using JavaScript
3.Ā Rock Paper Scissor Game using JavaScript
4.Ā Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeĀ
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- Font Awesome Icons --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA==" crossorigin="anonymous" /> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Random Quotes Generator using JavaScript with Source Code - @code.scientist x @codingtorque</title> </head> <body> <-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag
<div class="container"> <h2>Random Quote</h2> <p id="quote">"A thing well said will be wit in all languages." </p> <p id="author">- John Dryden</p> <button onclick="generateQuote()" class="generateBtn"><i class="fas fa-sync"></i></button> </div>
Output Till Now
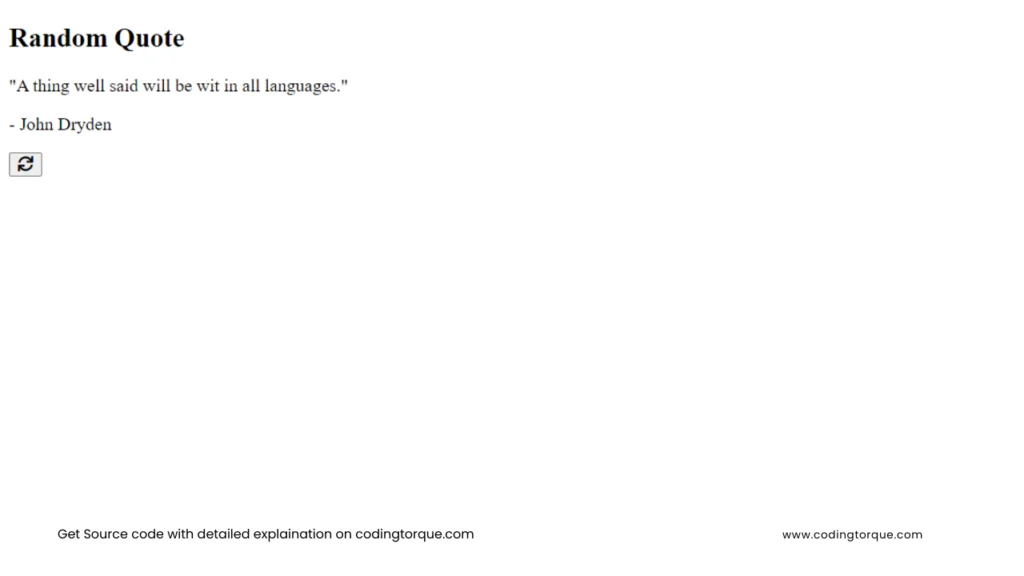
CSS CodeĀ
Create a fileĀ style.css
and paste the code below.
@import url("https://fonts.googleapis.com/css2?family=Poppins&family=Potta+One&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; font-family: "Poppins", sans-serif; } body { background: #171717; color: white; display: flex; align-items: center; justify-content: center; } .container { display: flex; align-items: center; justify-content: center; flex-direction: column; background-color: #262626; text-align: center; padding: 30px; width: 30rem; margin: 10rem; border-radius: 10px; } #quote { height: 6rem; margin-top: 20px; } .generateBtn { height: 50px; width: 50px; margin-top: 10px; border-radius: 50%; border: none; background: deepskyblue; color: white; cursor: pointer; font-size: 15px; }
Output Till Now
JavaScript CodeĀ
script.js
Ā and paste the code below.const generateQuote = () => { let url = "https://type.fit/api/quotes"; fetch(url).then(function (response) { return response.json() }).then(function (data) { let randNum = Math.floor((Math.random() * 1500) + 1); let randomQuote = data[randNum]; document.getElementById("quote").innerHTML = `"${randomQuote.text}"`; document.getElementById("author").innerHTML = `- ${randomQuote.author ? randomQuote.author : ""}`; }); }