Welcome to Coding Torque. In this blog, we are going to create a Background Color Switcher using HTML, CSS and JavaScript. You should create this project if you are a beginner and learning JavaScript.
Before we start, here are some JavaScript Games you might like to create:
1.Ā Snake Game using JavaScript
2.Ā 2D Bouncing Ball Game using JavaScript
3.Ā Rock Paper Scissor Game using JavaScript
4.Ā Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeĀ
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- Google Fonts --> <link rel="preconnect" href="https://fonts.googleapis.com"> <link rel="preconnect" href="https://fonts.gstatic.com" crossorigin> <link href="https://fonts.googleapis.com/css2?family=Poppins&display=swap" rel="stylesheet"> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>OnClick Background Color Change with Source Code - @code.scientist x @codingtorque</title> </head> <body> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag
<div class="container"> <h1>Background Color Switcher</h1> <div> <span class="button" id="grey"></span> <span class="button" id="deepskyblue"></span> <span class="button" id="blue"></span> <span class="button" id="yellow"></span> <span class="button" id="red"></span> <span class="button" id="green"></span> </div> </div>
Output Till Now
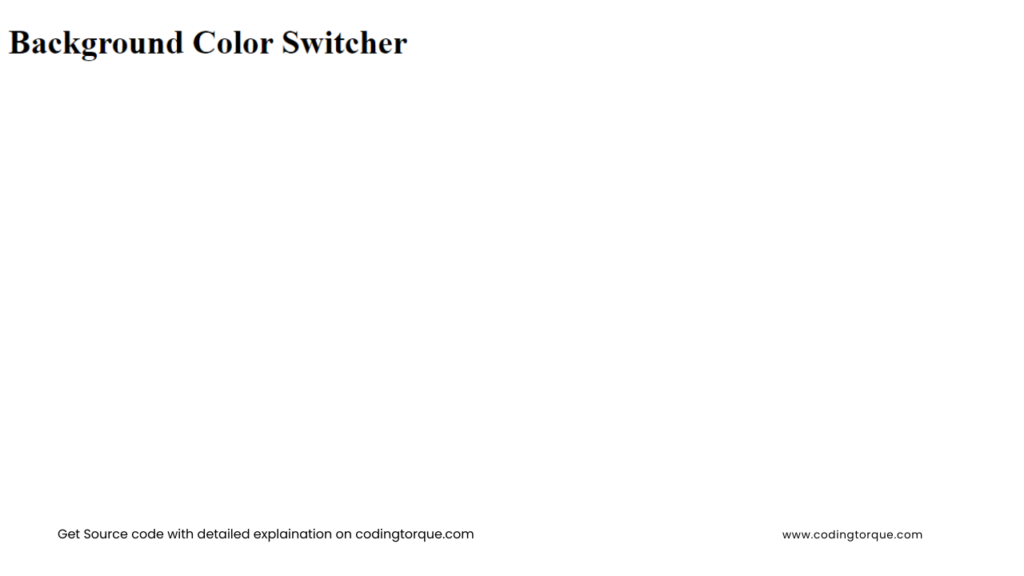
CSS CodeĀ
Create a fileĀ style.css
and paste the code below.
html { margin: 0; font-family: "Poppins", sans-serif; } body { display: flex; align-items: center; justify-content: center; margin-top: 15rem; } .container { background: white; width: 50rem; padding: 4rem 0; border-radius: 10px; display: flex; flex-direction: column; align-items: center; justify-content: center; } span { display: block; cursor: pointer; } .button { width: 100px; height: 60px; border: solid black 2px; display: inline-block; border-radius: 10px; } #grey { background: grey; } #deepskyblue { background: deepskyblue; } #blue { background: blue; } #yellow { background: yellow; } #red { background: red; } #green { background: green; }
Output Till Now
JavaScript CodeĀ
Create a fileĀ
script.js
Ā and paste the code below.const buttons = document.querySelectorAll('.button'); const body = document.querySelector('body'); console.log(buttons); buttons.forEach(function (button) { button.addEventListener('click', function (e) { console.log(e.target); if (e.target.id === 'grey') { body.style.backgroundColor = e.target.id; } if (e.target.id === 'deepskyblue') { body.style.backgroundColor = e.target.id; } if (e.target.id === 'blue') { body.style.backgroundColor = e.target.id; } if (e.target.id === 'yellow') { body.style.backgroundColor = e.target.id; } if (e.target.id === 'red') { body.style.backgroundColor = e.target.id; } if (e.target.id === 'green') { body.style.backgroundColor = e.target.id; } }) })
Written by: Piyush Patil
If you have any doubts or any project ideas feel free to Contact Us
Hope you find this post helpfulš