Welcome to Coding Torque, fellow coders! Are you looking to increase the security of your website or web application? In this tutorial, we will be creating a Captcha Generator using HTML, CSS, and JavaScript. Captchas, or Completely Automated Public Turing tests to tell Computers and Humans Apart, are a popular security measure used to protect websites from spam and automated bot attacks. By the end of this tutorial, you will have a working Captcha generator that you can easily implement on your own website. So, let’s get started and add an extra layer of protection to your online projects!
Before we start, here are some JavaScript Games you might like to create:
1.Ā Snake Game using JavaScript
2.Ā 2D Bouncing Ball Game using JavaScript
3.Ā Rock Paper Scissor Game using JavaScript
4.Ā Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeĀ
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- FONT AWESOME CDN --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.14.0/css/all.min.css" integrity="sha512-1PKOgIY59xJ8Co8+NE6FZ+LOAZKjy+KY8iq0G4B3CyeY6wYHN3yt9PW0XpSriVlkMXe40PTKnXrLnZ9+fkDaog==" crossorigin="anonymous" /> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Catpcha Generator using JavaScript - @code.scientist x @codingtorque</title> </head> <body onload="createCaptcha()"> <!-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag
<div class="container"> <div style="width:100%; display: flex; justify-content: space-between;"> <div id="captcha"> </div> <button class="regenerateCaptchaBtn" style="width: 5rem;" onclick="createCaptcha()"> <i class="fas fa-redo"></i> </button> </div> <input type="text" placeholder="Enter Captcha" id="cpatchaTextBox" /> <button onclick="validateCaptcha()">Submit</button> </div>
Output Till Now
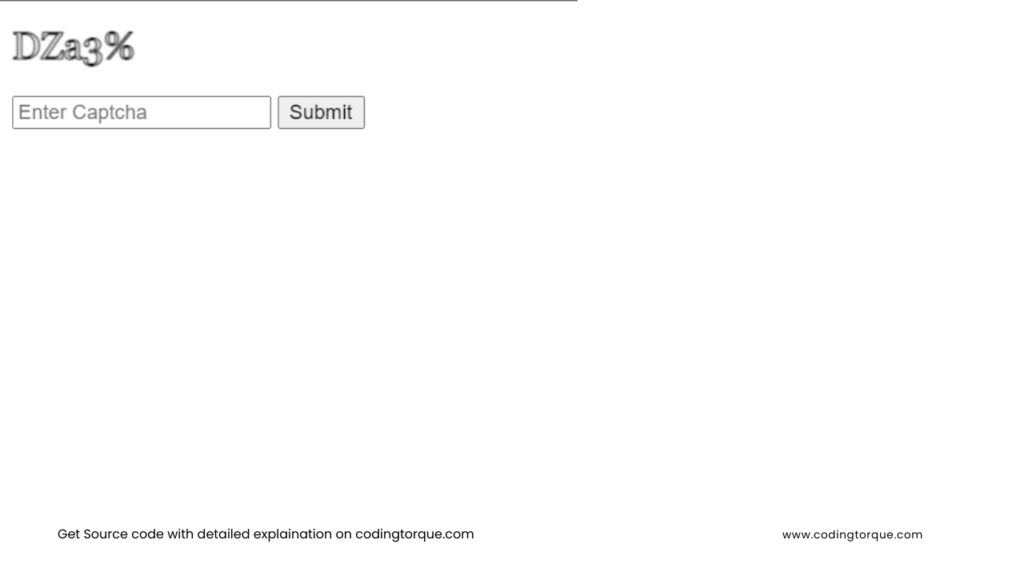
CSS CodeĀ
Create a fileĀ style.css
and paste the code below.
body { display: flex; align-items: center; justify-content: center; padding-top: 10rem; background-color: #2f66f5; } .container { width: 20rem; background: white; border-radius: 10px; padding: 2rem; display: flex; align-items: center; justify-content: center; flex-direction: column; box-shadow: 0 20px 25px -5px rgb(0 0 0 / 0.1), 0 8px 10px -6px rgb(0 0 0 / 0.1); } #captcha { border-radius: 5px; border: 1px solid gainsboro; display: flex; align-items: center; justify-content: center; } input[type="text"] { padding: 12px 20px; display: inline-block; border: 1px solid #ccc; border-radius: 5px; box-sizing: border-box; width: 100%; margin: 12px 0; } button { background-color: #2f66f5; border: none; color: white; padding: 12px 30px; text-decoration: none; margin: 4px 2px; cursor: pointer; width: 100%; border-radius: 5px; } canvas { pointer-events: none; }
Output Till Now
JavaScript CodeĀ
script.js
Ā and paste the code below.var code; function createCaptcha() { // clear the contents of captcha div first document.getElementById('captcha').innerHTML = ""; var charsArray = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ@!#$%^&*"; var lengthOtp = 6; var captcha = []; for (var i = 0; i < lengthOtp; i++) { //below code will not allow Repetition of Characters var index = Math.floor(Math.random() * charsArray.length + 1); //get the next character from the array if (captcha.indexOf(charsArray[index]) == -1) captcha.push(charsArray[index]); else i--; } var canv = document.createElement("canvas"); canv.id = "captcha"; canv.width = 100; canv.height = 50; var ctx = canv.getContext("2d"); ctx.font = "25px Georgia"; ctx.strokeText(captcha.join(""), 0, 30); //storing captcha so that can validate you can save it somewhere else according to your specific requirements code = captcha.join(""); document.getElementById("captcha").appendChild(canv); // adds the canvas to the body element } function validateCaptcha() { event.preventDefault(); debugger if (document.getElementById("cpatchaTextBox").value == code) { alert("Valid Captcha") } else { alert("Invalid Captcha. try Again"); createCaptcha(); } }