Welcome to Coding Torque! Are you ready to dive into the world of web development and build your very own Coin Toss Game using HTML, CSS, and JavaScript? This interactive and fun application is perfect for beginners looking to get started with front-end development. In this blog, we’ll walk you through the process of creating this simple yet engaging game from scratch. Whether you’re a seasoned coder or a complete beginner, you’ll find this tutorial helpful and enjoyable. So let’s get started!
Before we start, here are some JavaScript Games you might like to create:
1.Ā Snake Game using JavaScript
2.Ā 2D Bouncing Ball Game using JavaScript
3.Ā Rock Paper Scissor Game using JavaScript
4.Ā Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeĀ
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Coin Toss Game using JavaScript - @code.scientist x @codingtorque</title> </head> <body> <!-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag
<div class="container"> <div class="coin" id="coin"> <div class="heads"> <img src="https://upload.wikimedia.org/wikipedia/commons/0/0f/Indian_20_Rupee_coin.png?20211211151051"> </div> <div class="tails"> <img src="https://upload.wikimedia.org/wikipedia/commons/4/4e/Indian_20_Rupee_coin_Reverse.png?20211211151813"> </div> </div> <div class="stats"> <p id="heads-count">Heads: 0</p> <p id="tails-count">Tails: 0</p> </div> <div class="buttons"> <button id="reset-button"> Reset </button> <button id="flip-button"> Flip Coin </button> </div> </div>
Output Till Now
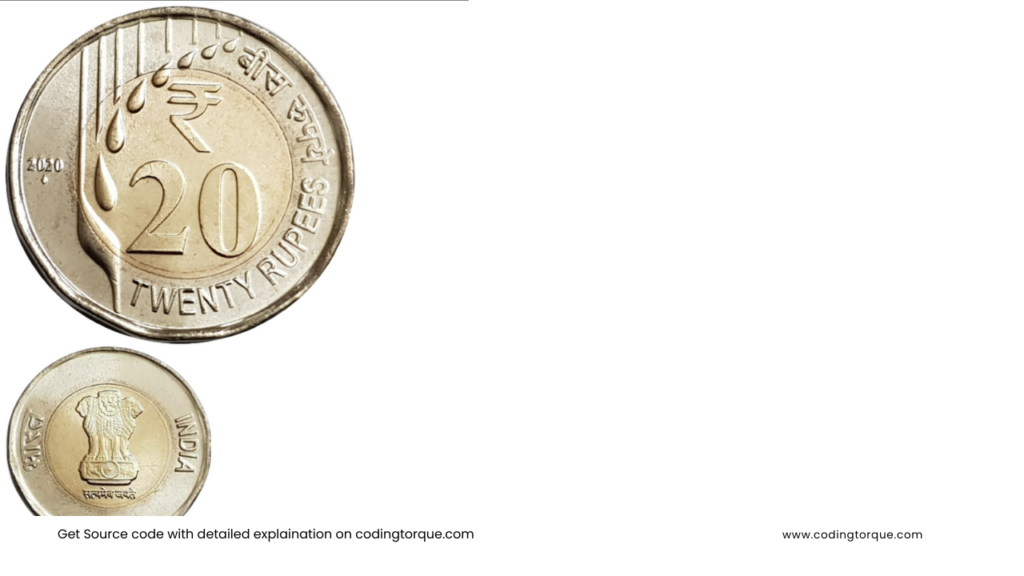
CSS CodeĀ
Create a fileĀ style.css
and paste the code below.
@import url("https://fonts.googleapis.com/css2?family=Poppins&display=swap"); * { padding: 0; margin: 0; box-sizing: border-box; font-family: "Rubik", sans-serif; } body { height: 100%; background: #4d59fb; } .container { background-color: #ffffff; width: 400px; padding: 35px; position: absolute; transform: translate(-50%, -50%); top: 50%; left: 50%; box-shadow: 15px 30px 35px rgba(0, 0, 0, 0.1); border-radius: 10px; -webkit-perspective: 300px; perspective: 300px; } .stats { display: flex; color: #101020; font-weight: 500; padding: 20px; margin-bottom: 40px; margin-top: 55px; border: 1px solid gainsboro; } .stats p:nth-last-child(1) { margin-left: 50%; } .coin { height: 150px; width: 150px; position: relative; margin: 32px auto; -webkit-transform-style: preserve-3d; transform-style: preserve-3d; } .coin img { width: 145px; } .heads, .tails { position: absolute; width: 100%; height: 100%; -webkit-backface-visibility: hidden; backface-visibility: hidden; } .tails { transform: rotateX(180deg); } @keyframes spin-tails { 0% { transform: rotateX(0); } 100% { transform: rotateX(1980deg); } } @keyframes spin-heads { 0% { transform: rotateX(0); } 100% { transform: rotateX(2160deg); } } .buttons { display: flex; justify-content: space-between; } button { width: 150px; padding: 15px 0; border: none; font-size: 16px; border-radius: 5px; cursor: pointer; } #flip-button { background-color: #4d59fb; color: #ffffff; } #flip-button:disabled { background-color: #e1e0ee; border-color: #e1e0ee; color: #101020; } #reset-button { background-color: #d39210; color: white; }
Output Till Now
JavaScript CodeĀ
script.js
Ā and paste the code below.let heads = 0; let tails = 0; let coin = document.querySelector(".coin"); let flipBtn = document.querySelector("#flip-button"); let resetBtn = document.querySelector("#reset-button"); flipBtn.addEventListener("click", () => { let i = Math.floor(Math.random() * 2); coin.style.animation = "none"; if (i) { setTimeout(function () { coin.style.animation = "spin-heads 3s forwards"; }, 100); heads++; } else { setTimeout(function () { coin.style.animation = "spin-tails 3s forwards"; }, 100); tails++; } setTimeout(updateStats, 3000); disableButton(); }); function updateStats() { document.querySelector("#heads-count").textContent = `Heads: ${heads}`; document.querySelector("#tails-count").textContent = `Tails: ${tails}`; } function disableButton() { flipBtn.disabled = true; setTimeout(function () { flipBtn.disabled = false; }, 3000); } resetBtn.addEventListener("click", () => { coin.style.animation = "none"; heads = 0; tails = 0; updateStats(); });