Are you ready to take your first step in the world of ReactJS? Curiosity is the key to unlocking your potential, and in this blog, we will use that curiosity to create a simple yet powerful food recipe app using ReactJS. Whether you’re a seasoned developer or just starting out, this blog is the perfect resource to guide you through the process of building your own React web app. You’ll learn how to leverage the power of React to create a user-friendly app that allows users to browse, search, and save their favorite recipes. With easy-to-follow instructions and helpful tips, you’ll be able to create a beautiful and functional app in no time. So, let’s harness the power of your curiosity and dive into the exciting world of ReactJS together!
Requirements:
1. Basic understanding of HTML, CSS, TailwindCSS, Javascript, and ReactJS.
2. NodeJS, NPM and create-react-app
.
3. Code Editor(I’m using VS Code).
Note: Before we start get your API Key from ( https://developer.edamam.com/ ). We are going to use EDAMAM to fetch all food recipes.
Now, let’s go step by step:
Step 1: Open your terminal and paste the below code to create react environment
npx create-react-app food-app
food-app is the name of my application you can give any name you want.
Step 2: After installation move to that folder by using following command.
cd food-app
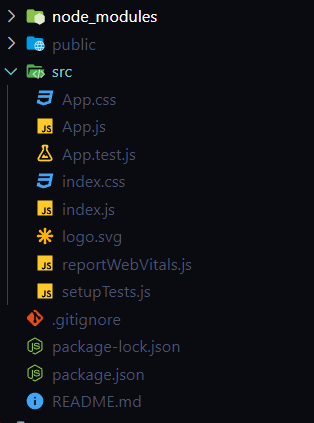
Step 3: Install tailwindCSS.
TailwindCSS is a CSS framework that makes quicker to write and maintain the code of your application.
Refer tailwindcss react guide to install tailwindcss in your application
Step 4: Now inside your src/App.js file replace the following code. Note that you have to replace <APPLICATION_KEY> and <APPLICATION_ID> which you have got from EDAMAM.
import React, { useEffect, useState } from 'react' import Recipe from './Recipe'; const App = () => { const APP_ID = "f643af62"; const APP_KEY = "2a8e753613ccb01b4d26d9716e1b971d"; const [recipes, setRecipes] = useState([]); const [search, setSearch] = useState(""); const [query, setQuery] = useState("chicken"); useEffect(() => { getRecipes(); }, [query]) const getRecipes = async () => { const response = await fetch (`https://api.edamam.com/search?q=${query}&app_id=${APP_ID}&app_key=${APP_KEY}`); const data = await response.json(); setRecipes(data.hits); }; const updateSearch = e => { setSearch(e.target.value); }; const getSearch = e => { e.preventDefault(); setQuery(search); setSearch(""); } return ( <div> <form class="text-gray-400 bg-gray-900 body-font" onSubmit={getSearch}> <div class="container py-10 mx-auto"> <div class="flex lg:w-1/2 w-full sm:flex-row flex-col mx-auto px-8 sm:px-0 items-end sm:space-x-4 sm:space-y-0 space-y-4"> <div class="relative sm:mb-0 flex-grow w-full"> <label for="full-name" class="leading-7 text-sm text-gray-400">Search Recipe</label> <input type="text" id="full-name" name="full-name" value={search} onChange={updateSearch} class="w-full bg-gray-800 bg-opacity-40 rounded border border-gray-700 focus:border-indigo-500 focus:ring-2 focus:ring-indigo-900 focus:bg-transparent text-base outline-none text-gray-100 py-1 px-3 leading-8 transition-colors duration-200 ease-in-out" /> </div> <button type="submit" class="text-white bg-indigo-500 border-0 py-2 px-8 focus:outline-none hover:bg-indigo-600 rounded text-lg">Search</button> </div> </div> </form> <section class="text-gray-600 body-font"> <div class="container px-5 py-24"> <div class="flex flex-wrap -m-4 w-4/5 mx-auto"> {recipes.map(recipe => ( <Recipe key={recipe.recipe.label} recipe={recipe.recipe} /> ))} </div> </div> </section> </div> ); } export default App
Step 5: Now create a component named Recipe.jsx in your src folder.
import React from "react"; const Recipe = ({ recipe }) => { return ( <div className="p-4 md:w-1/3"> <div className="h-full border-2 border-gray-200 border-opacity-60 rounded-lg overflow-hidden"> <img className="lg:h-48 md:h-36 w-full object-cover object-center" src={recipe.image} alt="blog" /> <div className="p-6"> <h2 className="tracking-widest text-xs title-font font-medium text-gray-400 mb-1"> {recipe.dishType[0]} </h2> <h1 className="title-font text-lg font-medium text-gray-900 mb-3"> {recipe.label} </h1> <p className="leading-relaxed mb-3"> <b>Ingredients: </b> {recipe.ingredientLines.map((ingredient)=>( <p>{ingredient}</p> ))} </p> <div className="flex items-center flex-wrap "> <a href={recipe.url} className="text-indigo-500 inline-flex items-center md:mb-2 lg:mb-0" > View Recipe </a> <span className="text-gray-400 mr-3 inline-flex items-center lg:ml-auto md:ml-0 ml-auto leading-none text-sm pr-3 py-1 border-r-2 border-gray-200"> 1.2K </span> <span className="text-gray-400 inline-flex items-center leading-none text-sm"> 6 </span> </div> </div> </div> </div> ); }; export default Recipe;
And yes we are done! Just type the following command to run the application.
npm start
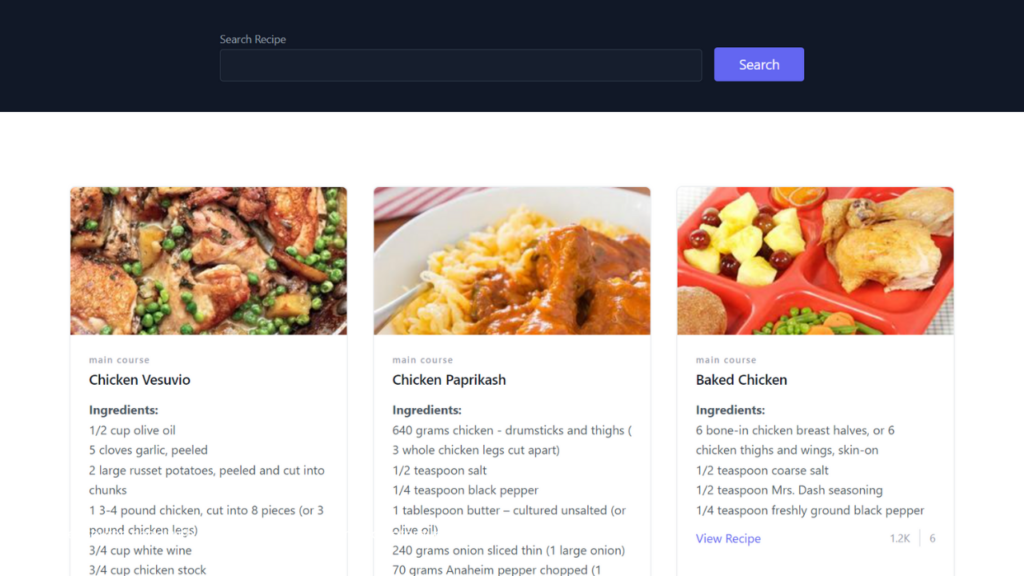