Step into the dynamic realm of Coding Torque, where innovation and ingenuity collide! Prepare yourself for an extraordinary journey as we dive headfirst into the creation of a cutting-edge Synonyms Search App, harnessing the combined power of HTML, CSS, and JavaScript. Say goodbye to ordinary vocabulary searches, for this app will be your linguistic companion, unleashing a world of synonyms at your fingertips, all wrapped in an intuitive and visually stunning interface. Whether you’re a coding enthusiast eager to expand your skill set or a language lover seeking a seamless way to enrich your lexicon, this tutorial is your gateway to the exciting fusion of technology and linguistics. So, tighten your seatbelts, as we embark on this thrilling quest, transforming the way you discover the nuances of language with the Synonyms Search App – where the magic of coding meets the art of expression!
Before we start here are 50 projects to create using HTML CSS and JavaScript –
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
Demo
HTML Code
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Synonyms Search App using HTML CSS and JavaScript - Coding Torque</title> </head> <body> <!-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag.
<div class="container"> <h1>Synonyms Search App</h1> <div class="search"> <input type="text" id="searchInput" placeholder="Enter a word"> <button id="searchButton"> <i class="fas fa-search"></i> </button> </div> <ul id="synonymsList"></ul> </div>
CSS Code
Create a file style.css and paste the code below.
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; font-family: "Poppins", sans-serif; } body { background-color: #e5e7eb; } .container { max-width: 40rem; margin: 0 auto; text-align: center; padding: 20px; margin-top: 10rem; } h1 { font-size: 24px; margin-bottom: 20px; } .search { display: flex; align-items: center; justify-content: center; border: 2px solid gainsboro; border-radius: 5px; overflow: hidden; background-color: white; box-shadow: 0 4px 6px -1px rgb(0 0 0 / 0.1), 0 2px 4px -2px rgb(0 0 0 / 0.1); } input[type="text"] { width: 100%; padding: 10px; border: none; outline: none; height: 100%; } button { border: none; cursor: pointer; height: 3rem; width: 3rem; transition: 0.3s ease; background-color: lawngreen; } button:hover { background-color: greenyellow; } ul { display: flex; list-style: none; align-items: start; flex-wrap: wrap; margin-top: 2rem; background-color: white; box-shadow: 0 4px 6px -1px rgb(0 0 0 / 0.1), 0 2px 4px -2px rgb(0 0 0 / 0.1); border-radius: 5px; padding: 10px; } li { margin: 5px; border: 2px solid gainsboro; border-radius: 5px; padding: 5px 12px; font-size: 14px; background-color: white; }
JavaScript Code
Create a file script.js and paste the code below.
const searchInput = document.getElementById('searchInput'); const searchButton = document.getElementById('searchButton'); const synonymsList = document.getElementById('synonymsList'); searchButton.addEventListener('click', searchSynonyms); function searchSynonyms() { const word = searchInput.value.trim(); if (word !== '') { fetchSynonyms(word); } else { clearResults(); } } function fetchSynonyms(word) { const apiUrl = `https://api.datamuse.com/words?rel_syn=${word}&max=10`; fetch(apiUrl) .then(response => response.json()) .then(data => { displaySynonyms(data); }) .catch(error => { console.log('An error occurred:', error); }); } function displaySynonyms(synonyms) { synonymsList.innerHTML = ''; if (synonyms.length === 0) { synonymsList.innerHTML = 'No synonyms found.'; return; } synonyms.forEach(synonym => { const listItem = document.createElement('li'); listItem.textContent = synonym.word; synonymsList.appendChild(listItem); }); } function clearResults() { synonymsList.innerHTML = ''; searchInput.value = ''; }
Final Output
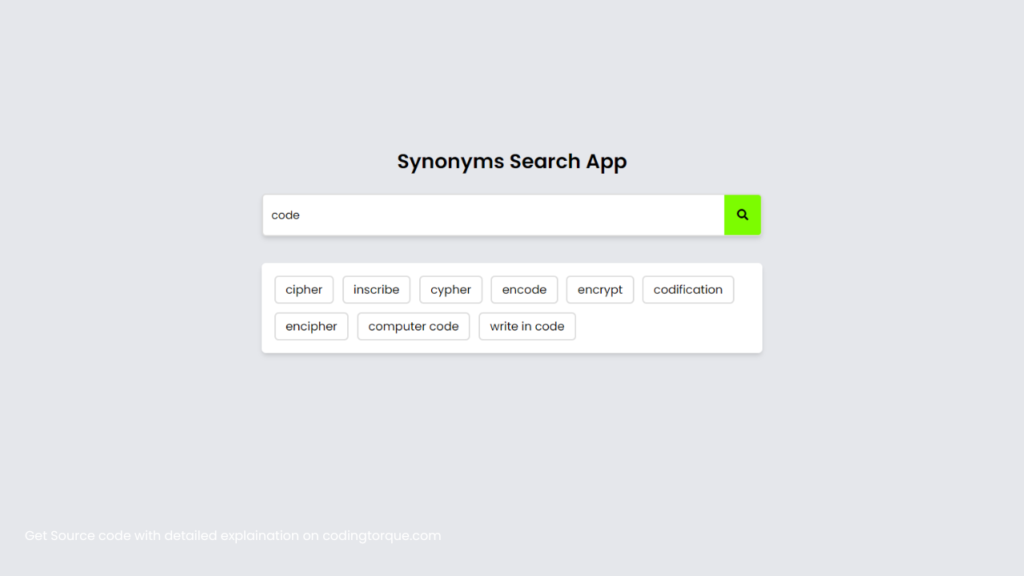
Written by: Piyush Patil
Code Credits: Piyush Patil
If you found any mistakes or have any doubts please feel free to Contact Us
Hope you find this post helpful💖