Hello Guys! Welcome to Coding Torque. In this blog, we are going to create a Custom Modal using HTML, CSS & JavaScript. You should create this project if you are a beginner and learning JavaScript. After creating this project you will be able to create a modal that displays the login/signup form, newsletter form, or any offer, etc.
Before we start, here are some JavaScript Games you might like to create:
1.Ā Snake Game using JavaScript
2.Ā 2D Bouncing Ball Game using JavaScript
3.Ā Rock Paper Scissor Game using JavaScript
4.Ā Tic Tac Toe Game using JavaScript
5. Whack a Mole Game using JavaScript
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
HTML CodeĀ
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- Font Awesome Icons CDN --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA==" crossorigin="anonymous" /> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Custom Modal using JavaScript with Source Code - @code.scientist x @codingtorque</title> </head> <body> <!-- Further code here --> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag
<!-- Trigger/Open The Modal --> <button id="openModalBtn" class="openModalBtn">Open Modal</button> <!-- The Modal --> <div id="modal" class="modal"> <!-- Modal content --> <div class="modal-content"> <div class="mHeading"> <p>Modal Heading</p> <span class="close"> <i class="fas fa-times"></i> </span> </div> <div class="mBody"> <p>Subscribe to our newsletter to get latest coding news and resources directly in your inbox.</p> <input type="email" placeholder="Enter your email address"> </div> <div> <button class="subscribeBtn">Subscribe</button> </div> </div> </div>
Output Till Now
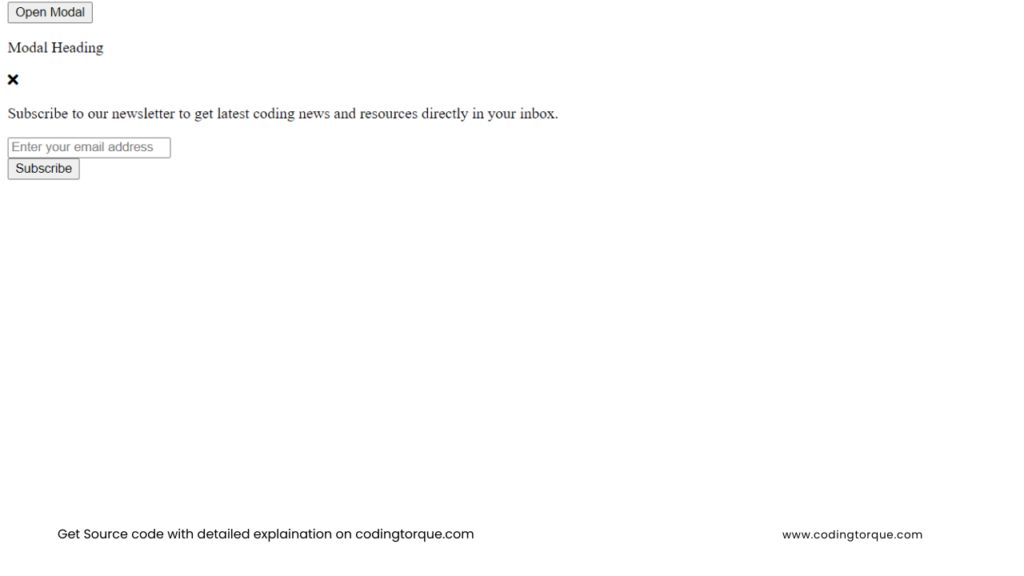
CSS CodeĀ
Create a fileĀ style.css
and paste the code below.
@import url("https://fonts.googleapis.com/css2?family=Poppins"); * { margin: 0; padding: 0; box-sizing: border-box; font-family: "Poppins", sans-serif; } body { font-family: Arial, Helvetica, sans-serif; display: flex; flex-direction: column; align-items: center; } .openModalBtn { background: black; color: white; padding: 10px 20px; border-radius: 10px; cursor: pointer; margin-top: 12rem; } .modal { display: none; position: fixed; z-index: 1; padding-top: 100px; left: 0; top: 0; width: 100%; height: 100%; overflow: auto; background-color: rgb(0, 0, 0); background-color: rgba(0, 0, 0, 0.4); } .modal-content { background-color: #fefefe; margin: auto; padding: 20px; border: 1px solid #888; width: 60%; border-radius: 10px; } .mHeading { display: flex; align-items: center; justify-content: space-between; padding-bottom: 10px; border-bottom: 2px solid; } .close { background-color: gainsboro; color: #aaaaaa; width: 30px; height: 30px; border-radius: 50%; display: flex; align-items: center; justify-content: center; font-size: 15px; transition: 0.3s ease; } .close:hover, .close:focus { color: white; background-color: black; text-decoration: none; cursor: pointer; } .mBody { font-size: 14px; margin: 20px 0; } .mBody input { width: 100%; margin-top: 10px; height: 40px; padding: 0 10px; border: 2px solid gainsboro; border-radius: 10px; outline: none; } .subscribeBtn { background: black; color: white; padding: 10px 20px; border-radius: 10px; cursor: pointer; width: 100%; text-transform: uppercase; letter-spacing: 1px; transition: 0.3s ease; } .subscribeBtn:hover { letter-spacing: 2px; }
Output Till Now
JavaScript CodeĀ
script.js
Ā and paste the code below.// Get the modal var modal = document.getElementById("modal"); var btn = document.getElementById("openModalBtn"); var span = document.getElementsByClassName("close")[0]; btn.onclick = function () { modal.style.display = "block"; } span.onclick = function () { modal.style.display = "none"; } window.onclick = function (event) { if (event.target == modal) { modal.style.display = "none"; } }