Are you a fan of minimalist design? Consider building a “Minimalist Clock” using HTML, CSS, and JavaScript. This elegant and simple design is perfect for those who prefer a clean and uncluttered aesthetic. In this tutorial, we’ll show you step-by-step how to create a “Minimalist Clock” from scratch, using only these three languages. You’ll learn how to create the clock face, add the hour and minute hands, and update the time using JavaScript. Plus, you’ll gain valuable experience in using CSS to create dynamic and responsive designs. By the end of this tutorial, you’ll have a functional and stylish “Minimalist Clock” that you can use as a website feature or as a desktop widget. So, let’s get started on creating a beautiful and minimalist clock using HTML, CSS, and JavaScript!
Before we start here are some more projects you might like to create –
- Duck Hunt Game using HTML and CSS
- 3D CSS Cards with Hover Effect
- IPhone X Design using HTML CSS and JavaScript
- CSS Glassmorphism Cards with Hover Effects
I would recommend you don’t just copy and paste the code, just look at the code and type by understanding it.
Demo
HTML Code
Starter Template
<!doctype html> <html lang="en"> <head> <!-- Required meta tags --> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- CSS --> <link rel="stylesheet" href="style.css"> <title>Minimalist Clock using HTML CSS and JavaScript - Coding Torque</title> </head> <body> <!-- Further code here --> <script src="https://code.jquery.com/jquery-3.6.4.js" integrity="sha256-a9jBBRygX1Bh5lt8GZjXDzyOB+bWve9EiO7tROUtj/E=" crossorigin="anonymous"> </script> <script src="script.js"></script> </body> </html>
Paste the below code in your <body>
tag.
<div class="clock"> <div class="clock__second"></div> <div class="clock__minute"></div> <div class="clock__hour"></div> <div class="clock__axis"></div> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> <section class="clock__indicator"></section> </div>
CSS Code
Create a file style.css and paste the code below.
html, body { height: 100%; } body { background: #1b1e23; display: flex; flex-direction: column; justify-content: center; align-items: center; font-family: sans-serif; color: white; } p { color: #f4eed7; font-size: 0.8em; opacity: 0.75; } .clock { height: 200px; width: 200px; border-radius: 100px; display: flex; justify-content: center; position: relative; } .clock__second, .clock__minute, .clock__hour, .clock__indicator { position: absolute; left: calc(50% - 1px); width: 2px; background: #f4eed7; transform-origin: bottom center; z-index: 2; border-radius: 1px; } .clock__second { height: 90px; margin-top: 10px; background: #4b9aaa; animation: time 60s infinite steps(60); z-index: 3; } .clock__minute { height: 80px; margin-top: 20px; opacity: 0.75; animation: time 3600s linear infinite; } .clock__hour { height: 60px; margin-top: 40px; animation: time 43200s linear infinite; } .clock__indicator { height: 98px; border-top: 2px solid #4b9aaa; background: none; } .clock__indicator:nth-of-type(5n) { opacity: 1; height: 93px; border-top: 7px solid #f4eed7; } .clock__axis { background: #4b9aaa; width: 5px; height: 5px; border-radius: 3px; position: absolute; z-index: 4; top: 97px; } section:nth-of-type(1) { transform: rotateZ(calc(6deg * 1)); } section:nth-of-type(2) { transform: rotateZ(calc(6deg * 2)); } section:nth-of-type(3) { transform: rotateZ(calc(6deg * 3)); } section:nth-of-type(4) { transform: rotateZ(calc(6deg * 4)); } section:nth-of-type(5) { transform: rotateZ(calc(6deg * 5)); } section:nth-of-type(6) { transform: rotateZ(calc(6deg * 6)); } section:nth-of-type(7) { transform: rotateZ(calc(6deg * 7)); } section:nth-of-type(8) { transform: rotateZ(calc(6deg * 8)); } section:nth-of-type(9) { transform: rotateZ(calc(6deg * 9)); } section:nth-of-type(10) { transform: rotateZ(calc(6deg * 10)); } section:nth-of-type(11) { transform: rotateZ(calc(6deg * 11)); } section:nth-of-type(12) { transform: rotateZ(calc(6deg * 12)); } section:nth-of-type(13) { transform: rotateZ(calc(6deg * 13)); } section:nth-of-type(14) { transform: rotateZ(calc(6deg * 14)); } section:nth-of-type(15) { transform: rotateZ(calc(6deg * 15)); } section:nth-of-type(16) { transform: rotateZ(calc(6deg * 16)); } section:nth-of-type(17) { transform: rotateZ(calc(6deg * 17)); } section:nth-of-type(18) { transform: rotateZ(calc(6deg * 18)); } section:nth-of-type(19) { transform: rotateZ(calc(6deg * 19)); } section:nth-of-type(20) { transform: rotateZ(calc(6deg * 20)); } section:nth-of-type(21) { transform: rotateZ(calc(6deg * 21)); } section:nth-of-type(22) { transform: rotateZ(calc(6deg * 22)); } section:nth-of-type(23) { transform: rotateZ(calc(6deg * 23)); } section:nth-of-type(24) { transform: rotateZ(calc(6deg * 24)); } section:nth-of-type(25) { transform: rotateZ(calc(6deg * 25)); } section:nth-of-type(26) { transform: rotateZ(calc(6deg * 26)); } section:nth-of-type(27) { transform: rotateZ(calc(6deg * 27)); } section:nth-of-type(28) { transform: rotateZ(calc(6deg * 28)); } section:nth-of-type(29) { transform: rotateZ(calc(6deg * 29)); } section:nth-of-type(30) { transform: rotateZ(calc(6deg * 30)); } section:nth-of-type(31) { transform: rotateZ(calc(6deg * 31)); } section:nth-of-type(32) { transform: rotateZ(calc(6deg * 32)); } section:nth-of-type(33) { transform: rotateZ(calc(6deg * 33)); } section:nth-of-type(34) { transform: rotateZ(calc(6deg * 34)); } section:nth-of-type(35) { transform: rotateZ(calc(6deg * 35)); } section:nth-of-type(36) { transform: rotateZ(calc(6deg * 36)); } section:nth-of-type(37) { transform: rotateZ(calc(6deg * 37)); } section:nth-of-type(38) { transform: rotateZ(calc(6deg * 38)); } section:nth-of-type(39) { transform: rotateZ(calc(6deg * 39)); } section:nth-of-type(40) { transform: rotateZ(calc(6deg * 40)); } section:nth-of-type(41) { transform: rotateZ(calc(6deg * 41)); } section:nth-of-type(42) { transform: rotateZ(calc(6deg * 42)); } section:nth-of-type(43) { transform: rotateZ(calc(6deg * 43)); } section:nth-of-type(44) { transform: rotateZ(calc(6deg * 44)); } section:nth-of-type(45) { transform: rotateZ(calc(6deg * 45)); } section:nth-of-type(46) { transform: rotateZ(calc(6deg * 46)); } section:nth-of-type(47) { transform: rotateZ(calc(6deg * 47)); } section:nth-of-type(48) { transform: rotateZ(calc(6deg * 48)); } section:nth-of-type(49) { transform: rotateZ(calc(6deg * 49)); } section:nth-of-type(50) { transform: rotateZ(calc(6deg * 50)); } section:nth-of-type(51) { transform: rotateZ(calc(6deg * 51)); } section:nth-of-type(52) { transform: rotateZ(calc(6deg * 52)); } section:nth-of-type(53) { transform: rotateZ(calc(6deg * 53)); } section:nth-of-type(54) { transform: rotateZ(calc(6deg * 54)); } section:nth-of-type(55) { transform: rotateZ(calc(6deg * 55)); } section:nth-of-type(56) { transform: rotateZ(calc(6deg * 56)); } section:nth-of-type(57) { transform: rotateZ(calc(6deg * 57)); } section:nth-of-type(58) { transform: rotateZ(calc(6deg * 58)); } section:nth-of-type(59) { transform: rotateZ(calc(6deg * 59)); } section:nth-of-type(60) { transform: rotateZ(calc(6deg * 60)); } @keyframes time { to { transform: rotateZ(360deg); } }
JavaScript Code
Create a file script.js and paste the code below.
var currentSec = getSecondsToday(); var seconds = (currentSec / 60) % 1; var minutes = (currentSec / 3600) % 1; var hours = (currentSec / 43200) % 1; setTime(60 * seconds, "second"); setTime(3600 * minutes, "minute"); setTime(43200 * hours, "hour"); function setTime(left, hand) { $(".clock__" + hand).css("animation-delay", "" + left * -1 + "s"); } function getSecondsToday() { let now = new Date(); let today = new Date(now.getFullYear(), now.getMonth(), now.getDate()); let diff = now - today; return Math.round(diff / 1000); }
Final Output
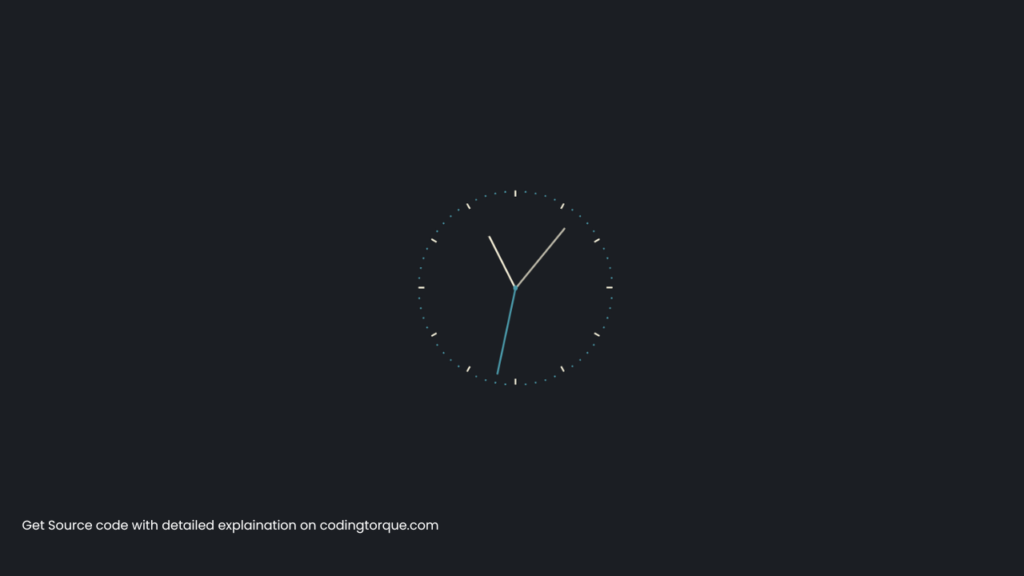
Written by: Piyush Patil
Code Credits: @kylewetton
If you found any mistakes or have any doubts please feel free to Contact Us
Hope you find this post helpful💖